Here we will analyze template of the user interface (created in the lesson 7), clear it from unnecessary code, so in the future you will be able to use the template to write your demo applications.
Now, knowing the components of user interface, let's take a look at the standard template that we had created in the lesson 7.
Let's start with the window (Win). Win is the root element of the screen. It will be the parent for the other components. To create the window, use the function:
elm_win_util_standard_add(const char *name, const char *title);
This function is derivative of the function:
elm_win_add(Evas_Object *parent, const char *name, Elm_Win_Type type);
which creates a standard window with the specified name. The difference between the first function and the second one is that it creates a window with an already added background widget and assigns a title to the window. However, these two parameters are not relevant for the Wearable profile; they can be ignored by passing into the function: (NULL, NULL). For a standard window, the parent is not required, so this parameter is NULL. The following function specifies that the window will not contain the conformant:
elm_win_conformant_set(ad->win, EINA_TRUE);
Option:
elm_win_autodel_set(ad->win, EINA_TRUE);
means that the window object will be automatically deleted when the application is closed. So to free resources, there is no need to explicitly invoke window deletion. If the function elm_win_wm_rotation_supported_get (ad-> win) returns true, it means that the window manager supports window rotation. Also you can specify for a window manager how to rotate the window. Next lines give command to the manager to rotate the window by 90 °. The number of available turns is 4.
if (elm_win_wm_rotation_supported_get(ad->win))
{
int rots[4] = {0, 90, 180, 270};
elm_win_wm_rotation_available_rotations_set(ad->win, (const int *)(&rots), 4);
}
Thus, for a full circle the window can change its orientation to 0°, 90°, 180° and 270°. As for the watch, the screen can be rotated only if they are on a stand, during charging. The window object, like the most of other screen components objects, has the Evas_Object type. For all such objects can be registered or added callback functions that handle various events with which we will introduce you gradually. The first method we'll look at is a handler of window deletion function. Please note that the function:
evas_object_smart_callback_add(ad->win,
"delete,request",
win_delete_request_cb,
NULL);
presented in the template, is not suitable for the application written for the Wearable device. Since when user presses the system "X" button - close, which is located on the panel of the window, this smart callback is triggered in the desktop version of EFL applications. Most likely, this function was inadvertently copied from the enlightenment of the EFL documentation for desktop applications. Therefore, you should be more careful with examples presented at developer.tizen.org.
Function:
eext_object_event_callback_add(ad->win, EEXT_CALLBACK_BACK, _win_back_cb, ad);
registers the handler function for pressing hardware Back button.
The callback has following signature:
typedef void (*Eext_Event_Cb)(void *data, Evas_Object *obj, void *event_info);
To minimize the window, click the Back button.
static void
win_back_cb(void *data, Evas_Object *obj, void *event_info)
{
appdata_s *ad = data;
/* Let window go to hide state. */
elm_win_lower(ad->win);
}
If you need not just to minimize the window, but to close the application, you may call the function ui_app_exit(), which interrupts the main loop of the application and it ends its work.
It is container from the Elementary library. The conformant consists of several parts: content (occupies all space; in this area will be placed user interface), system indicator (superimposed on the content) and virtual keyboard. Thus, if you use the system keyboard in your application, you can be sure that it will be displayed on top of your entire user interface. The diagram below shows the layout of the conformant.
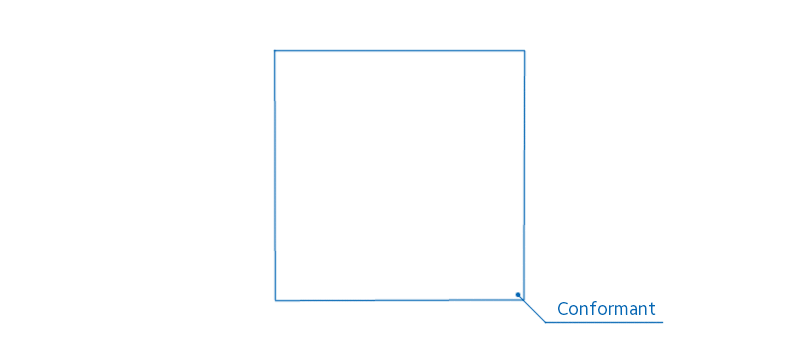
The area for the indicator is not used in the Wearable profile, which is probably due to the small size of the screen. The content area can be filled using the elm_object_content_set (...) method. To create conformant use following function:
ad->conform = elm_conformant_add(ad->win);
We can also give recommendations on the size of the conformant. The following function prescribes that the conformant will occupy all the allocated space (horizontally and vertically).
evas_object_size_hint_weight_set(ad->conform,
EVAS_HINT_EXPAND,
EVAS_HINT_EXPAND);
Line:
elm_win_resize_object_add(ad->win, ad->conform);
means that the window determines the size of the conformant, so the dimensions of the conformant change to match the window size. To display the conformant use the following function:
evas_object_show(ui->conform);
Label — is a widget for displaying text. To format the text, this widget uses an HTML-like markup language. Use lines below to create a label. In the center of the widget, add text: "Hello Tizen". Further, it is indicated that the widget supposed to fill all the space provided to it. In this case, it is the content area of the conformant, since label is added as the content of the conformant.
ad->label = elm_label_add(ad->conform);
elm_object_text_set(ui->label, "Hello Tizen");
evas_object_size_hint_weight_set(ad->label, EVAS_HINT_EXPAND, EVAS_HINT_EXPAND);
elm_object_content_set(ad->conform, ad->label);
At first, you should display all the objects included in the window and only then - window itself.
evas_object_del(ad->win);
In the documentation it is strongly recommended to display the window only after the entire base GUI was created. This method is more productive, because it allows you to avoid performing unnecessary system operations, at the start of the application.
Below you can see the reformatted code from the application template, so in the following lessons it will be more convenient to use it during creations of different demo applications. The header file was deleted from the project, now all the initial code is in the main.c file. Also, to make code clearer some comments were added. The edited code is shown below; also it is available for downloading here WearUITemplate.
// Functions for managing the main application cycle,
// registering the callbacks
// for application creation and termination
#include <app.h>
// Functions for creating and managing user interface elements
// There are all the widgets, such as: windows, buttons, etc.
#include <Elementary.h>
// Functions for using callbacks for the hardware Back button and for
// using extended widgets for a round screen
#include <efl_extension.h>
typedef struct _UIData {
Evas_Object *win;
Evas_Object *conform;
} UIData;
static void
_win_back_cb(void *data, Evas_Object *obj, void *event_info)
{
UIData *ui = data;
elm_win_lower(ui->win);
}
static void
_conformant_create(UIData *ui)
{
ui->conform = elm_conformant_add(ui->win);
// Specify to the conformant to occupy all space allocated to it
// horizontally and vertically
evas_object_size_hint_weight_set(ui->conform, EVAS_HINT_EXPAND, EVAS_HINT_EXPAND);
evas_object_show(ui->conform);
}
static void
_window_create(UIData *ui)
{
// Create and configure the main window
ui->win = elm_win_util_standard_add(NULL, NULL);
// Create a conformant - in this case it is the main container
// in the application,
// which is also the interface between our application and
// system elements, such as the keyboard
_conformant_create(ui);
// Set the size of the conformant widget same as the window size
elm_win_resize_object_add(ui->win, ui->conform);
// Register the function that will be called
// when the hardware button Back is pressed
eext_object_event_callback_add(ui->win, EEXT_CALLBACK_BACK, _win_back_cb, ui);
// Always display the window only after the entire base of
// user interface was displayed.
evas_object_show(ui->win);
}
static bool
_app_create_cb(void *data)
{
UIData *ui = data;
_window_create(ui);
return true;
}
static void
_app_terminate_cb(void *data)
{
UIData *ui = data;
// Before the application finishes, delete the main widget (window),
// then all widgets-children of this window (all widgets of your application)
//will be deleted.
evas_object_del(ui->win);
}
int
main(int argc, char *argv[])
{
UIData ui = {0,};
ui_app_lifecycle_callback_s lifecycle_callbacks = {0,};
// Register the functions we have created to transfer them to the main loop
// of our application.
lifecycle_callbacks.create = _app_create_cb;
lifecycle_callbacks.terminate = _app_terminate_cb;
// Calling the next function, starts the execution of the main
// application loop
return ui_app_main(argc, argv, &lifecycle_callbacks, &ui);
}